Digital technologies/Arduino/Arduino- Beginner
Understanding Arduino Boards
What is a Micro-controller?
A micro-controller is a compact integrated circuit that receives input from the environment, processes the input and can produce an output. It receives input from its I/O pins and processes the signals received using the CPU onboard the chip. Micro-controllers are usually embedded in larger systems and are utilized in many areas of life including vehicles, medical devices, home appliances, and more.
Arduino is an open source electronics platform that provides an easy and accessible way to make robotics projects. The boards are able to receive input signals from sensors and can produce outputs through I/O pins. Arduino boards are used by a diverse set of people, including students, hobbyists, engineers, researchers due to the simple layout and programmability of the Arduino boards.
An Arduino or microcontroller can be implemented in many ways:
Arduino board sections
There are 5 important board sections that the user must have a solid understanding of to start utilizing the board's functions. These sections are outlined below:
Section | Description |
---|---|
USB connector | The arduino can be powered through a type A/B USB connector from the user’s laptop/computer to the board. This port is also used to upload programs onto the board. |
Power port | The arduino board can be powered through an AC-DC adapter or a battery. The power jack of the board can be connected to a 2.1 mm center-positive plug. |
Power pins | To power external circuitry, 3 standard voltages (0 V or GND, 3.3V, 5V) are provided in the ‘Power Pins’ section of the board. |
Digital pins | Digital pins on the arduino board can be configured as an input or an output. When the pins are configured as an input, the pins will send a binary signal into the board, which enables the board to read the sensed logic voltage levels (ie. either 0/low or 1/high). If the digital pins are configured as an output, then the arduino will send a binary signal to the pin. There is a built-in LED pre-connected to digital pin 13. When the value of the pin is driven HIGH by the processor, the LED on the board is illuminated, when the pin is LOW, it's turned off. This can be used as a status indicator when programs are running. |
Analog pins | The analog pins allow the arduino board to receive or send an analog signal. These signals need to be converted into digital representations which can be used inside the software-executing portion of the Arduino processor (which only uses binary digital signals). Analog signal inputs can be accepted for conversion into digital via the Analog Pins header. The Analog to Digital (A/D or “A to D”) conversion is done inside the processor with specialized circuitry. |
Analog VS Digital signals
A signal is an electromagnetic or electric current that transfers information from one source to another. There are two main types of signals used in electronics: analog or digital signals.
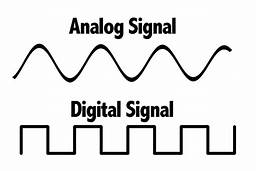
Analog signals
This is a time-varying and continuous type of signal that is often used to measure changes in light, sound, position, temperature, or other physical phenomena. When plotted in a voltage-time graph, the result is often a continuous and smooth curve.
Digital signals
A digital signal is a signal that represents information as a series of discrete binary values. Digital signals are used in all modern electronic applications, including communication and network devices. When plotted in a voltage-time graph, the signal is discrete, and ranges from 0 V to VCC (usually 1.8V, 3.3 V, or 5V).
Connecting to an Arduino
- There are a few ways that you can ruin or burn your Arduino, refer to this guide before starting for more information.
- If you are using a lab computer in the Faculty of Engineering refer to this guide for additional steps to connect the Arduino.
Step 1: Download the Arduino IDE from https://www.arduino.cc/en/software. Make sure to choose the version that is appropriate for your operating system.
Step 2: Connect the Arduino to your computer via an A/B type USB cable.
Step 3: Once the Arduino is connected, your computer will recognize the Arduino board as a generic COM port. The power LEDs onboard the Arduino should light up.
Step 4: Time to find out what port number is assigned so that the Arduino and computer can properly communicate with one another. in the IDE select Tools > Ports > Select a port. If even after selecting the port the Arduino doesn't connect, try a different port.
Step 5: Open the Arduino IDE. Under Tools>Board, ensure that the correct type of Arduino board is selected.
Step 6: Under Tools> COM, ensure that the “port” is the one shown in the step above. Check that the programmer is set as “AVRISP AKII”
Possible bugs:
If you're having trouble with your Arduino, refer to this guide: Arduino troubleshooting
Arduino IDE and Tools
Arduino IDE:
The process of programming includes designing and executing code in an integrated development environment, otherwise known as an IDE. Many different IDEs exist and are adopted for different usages, and allow programs to edit, debug, and execute (or compile) their code. In order to program an Arduino (regardless of what kind of microcontroller is used), one must have the Arduino IDE downloaded (Refer to Connecting an Arduino).
When beginning your journey in learning how to code, its important to get to know the integrated development environment, or IDE that the coder will use to edit and compile the written programs. The following figure guides the users on the basic options available on the IDE:
Internal Libraries
What is a library?
A library is a file that contains pre-written code that can be referred to in order to use certain sensors and functions. Often, in order to complete a task like connecting to a server to spinning a motor many lines of code must be written and executed. Libraries are bits of code that users can refer to in order to complete those tasks without having to type out each line of code, it simplifies the programming process and allows us to perform relatively complex tasks with ease.
In beginner topics we won’t be using complex or external libraries! But keep this in mind for the following ones as this will be necessary later on.
Built-in Libraries
Built-in libraries are ones that come preinstalled into the Arduino IDE, you don’t need to import the library into the IDE. An example of this type of library is the math.h library, which is used for most basic math operations.
Where to find them?
There are a few ways to look at what built-in libraries are available, you can do a quick search in different online Arduino forums, or the easiest way is to look at the library manager in the Arduino IDE. This window displays libraries that are already installed and you also have the ability to install new libraries that would would like.
As seen below, in the menu bar at the top of the window click on Tools > Manage Libraries...
Inside the manager as seen below you are able to select the type of library you are looking for, for example, installed, etc.
When wanting to use a library, one must declare it at the top of their code so that their IDE knows that that file is being referred to, the syntax is:
#include<math.h>
Serial Monitors
The serial monitor is a function in the Arduino IDE that allows you to interact with your Arduino. Through it you are able to send information and also receive feedback or the system output, this aids in debugging and interacting with the program.
The serial monitor can be accessed in two locations: the icon in the bar at the top of the IDE, as seen in the figure below.
And in the following drop down menu as shown below:
A few features to note is the baud rate and auto scroll functionality. The baud rate must match the one dictated in the program, this value dictates that rate that the Arduino and IDE communicate with each other.
When using the serial monitor it must be initialized in the code for a specific baud rate as follows:
Void Setup {
Serial.begin(9600); //this initializes the monitor at a baud rate of 9600 }
There are a few different ways to output values on to the serial monitor for example when wanting to output the value held by a variable the following syntax can be used:
Serial.print(variable_name):
Serial.println(variable_name); //for outputting each value on a new line
Alternatively, when wanting to output a string or phrase the following syntax can be used:
Serial.print("INSERT PHRASE");
Serial.println("INSERT PHRASE"); //for outputting each value on a new line
Introduction to Programming Syntax and Conceptualization
As mentioned previously, and IDE is used to compile and execute the code utilized in a microcontroller. There are many different IDEs that can be used for various types of microcontroller and for each purpose there are more suitable boards that can be used. In order to program an Arduino, one must have the Arduino IDE downloaded (Refer to Connecting an Arduino). The Arduino IDE provides users with a programming editor as well as a way to easily upload and compile programs onto the Arduino board. Programs in the Arduino IDE are called sketches, and are normally saved with the .ino extension. The language used to program the Arduino board is based on the C++ language, which is a general use Object Oriented language. Like any common language, in order to start coding, one must be aware of the grammar rules and vocabulary that is used. An important word that will be often encountered is a “function”, which is a block of code that takes in an input, processes the input, then returns an output.
Blink program: An Example
The Arduino IDE provides creators with a plethora of written programs that are fully ready to run on an Arduino board. They are located in the Files>Examples folder. Among the most basic is the "Blink" program, which can be used to not only get to know the basic features in the software and the hardware, but are also a great way to test the connectivity between the Arduino board and the user's computer. This program is located in Files>Examples>01.Basics>Blink.
The following provides an overview of the different functions used in this program:
Function | Description |
---|---|
Setup() | Performs any actions that are initially required to run the rest of the program, such as initializing any peripheral components and setting the communication frequency between the Arduino and the PC. |
Loop() | The loop function acts as the program's driver, it runs on a continuous loop and specifies the order of operation the microcontroller will perform. Execution starts at the top, goes through the contents of the loop and then starts executing from the top again. This procedure is repeated forever. |
pinMode (pin number, INPUT or Output) | Configures the pin to behave as either an INPUT or an OUTPUT. |
digitalWrite (pin number, HIGH or LOW) | Writes a HIGH or LOW value to a digital pin. If the pin has been configured as an OUTPUT, then the signal sent over (ie the voltage) will be set as 5 V (HIGH), or 0 V (LOW). For 3.3 V output boards, the high value will be set to 3.3 V, whereas the low is the same as the 5V board, which is 0V. |
Delay (time in milliseconds) | Pauses the program for the amount of time specified in the parameter. |
Initializing a Pin as an Input or Output
When using components you will often have to initialize the pin they are connected to as either an input or output! The syntax for this will vary depending on the component and what pin type it is connected to.
Analog Input/output
When trying to manipulate data from an analog pin, the following lines of code are used to read data from it:
int variable = analogRead(insert pin number); //here a variable is being defined to hold the value read from the pin
When you want to write data to the pin the following syntax is used:
int variable = analogWrite(insert pin number); //here a variable is being defined to hold the value read from the pin
Digital Input/output
When wanting to use a digital pin you will need to declare as either an input or output in the Void Setup () as follows:
pinMode(LED1, OUTPUT); //For example if you had a pin called LED1
When wanting to write a value to LED1 then you would use the following syntax:
digitalWrite(LED1, HIGH); //Here HIGH or LOW would indicate if you would like the led to turn on - for turning it on you set HIGH, and LOW otherwise.
Some other basic considerations:
Symbol | Description | |
---|---|---|
Brackets | {....} | Starts and ends a function or is used to group different statements together |
Comment bars | /* ….*/ or // | Allows coders to add comments to their code to make it more readable to other humans. Important to note that all comments do not get executed by the program and therefore do not alter the program! |
Semicolon | ...; | This character ends a program statement and lets the compiler know ‘the end of the current line/statement’ |
It is also important to be aware that the Arduino editor is case sensitive, meaning that the words “DOOR” and “Door” are not understood to be the same word by the compiler. Furthermore, to make writing and editing code more friendly, the Arduino IDE will colour code important functions, comments, etc. This will be seen later in this section.
As seen below, certain preexisting functions in the Arduino IDE will often times be a certain colour. This is a good indicator of if whether or not you have written the function correctly.
Pseudocode and Flowcharts
As the complexity of your projects progress keeping track of all the different functions will likely become increasingly more difficult. Some tools that are often used to help create a layout of what the purpose of the programs is include pseudocode and flowchart. They both aid in visualizing and outlining the logic behind your code, the difference between the two is that flowcharts are more visually based and in contrast pseudocode is a typed-up outline of the code [refer to examples below].
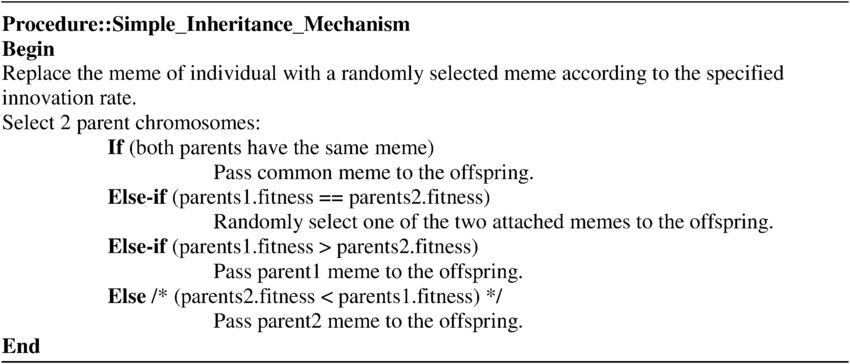
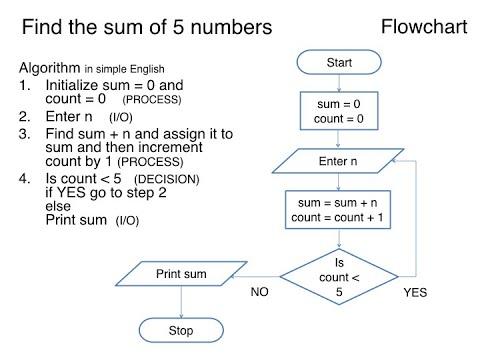
This allows the designer (you) to be able to work through the logic behind the code before creating it, allowing for a far smoother design process.
Introduction to Variables and Conditional Statements
Variables
Variables allow information in programmers to be stored or changed within the code. In order to create a variable within your program, it must be declared. To declare a variable, the coder has to write the type of variable to be declared first. Different types exist, most commonly used are:
Type | Syntax |
---|---|
Integers | int |
non-integers (rational and non-rational) | double or float |
characters | char |
After declaring the variable type, the coder must then name the variable. The variable name should clearly reflect the purpose of the value, so that the coder and the reader can easily identify which value is associated with what variable. Additionally, variables cannot have spaces embedded within the name. For example, "My deposit" is not a valid variable name, but "MyDeposit" is. Note that capitalization can be useful for readability. Furthermore, variables should not start with digits, or with an underscore "_".
After naming the variable, the coder can choose to initialize the variable, which is choosing an initial value to be associated with the variable (which can change throughout the program). Be careful to stay consistent with the type of the variable. For example, you cannot initialize the variable int Age with a value of "k", as "k" is not an integer. The code segments below illustrate the process of initialization:
int a;// not initialized, which is ok!
double b=1.23;/* Note that the 1.23 value matches with the “double” type which is a rational number*/
char MyVariable = “h”; //note the semicolons!
The following will produce an error, which will disable the compiler from compiling your code:
char YourVariable= 1.23; // 1.23 is not a character!
Variables can be classified into either global or local variables. The main difference is the scope at which they can be accessed. A global variable can be accessed by all functions that exist within a program, whereas a local variable can be accessed through only the function where it is declared. The example below illustrates this well [https://www.arduino.cc/reference/en/language/variables/variable-scope-qualifiers/scope/]:
/* There are two functions in this program: setup() and loop(), and three variables: "k", "i", "f".*/
int k; // any function will see this variable, thus called a global variable.
void setup() {
// ... }
void loop() {
int i; // "i" is only "visible" inside of "loop"
float f; } // "f" is only "visible" inside of "loop"
.....// some lines of code}
Conditionals
Conditional statements are programming operations that tells the computer to perform an action for a certain set of conditions. An "if" statement is the most common, and tells the computer to perform a set of actions only if the condition is fulfilled. The syntax is the following:
if (condition) {
/// a set of instructions }
Common alteration of the if statements are the "if...else" and "if... else if". The "if...else" conditionals first checks the "if" conditional, in the case that its true, the compiler will ignore the "else" set of instructions. In the case that the condition(s) declared in the "if" statement are not fulfilled, the "else" instructions will automatically run. The syntax for an "if...else" statement is:
if (condition){
///instructions;}
else {
///instructions that will ONLY run in the case the "if" conditions are not fulfilled }
In the case of the "if...else if" set of instructions, the program will only run if the conditions specified in the "if" or "else if" are true. The syntax is the following:
if (condition){
///instructions }
else if (condition) {
///instructions }
Introduction to Electronics and Circuitry
Introduction to Circuits
A circuit is a pathway where electricity can flow through a closed path from the negative (cathode) to the positive (anode) end of a power source. Elements in a circuit can either supply or expend energy. Energy is measured in Joules (J). Suppliers of energy are called sources; they provide a voltage, or charge electrons with energy. The number of electrons flowing in a circuit is called a current, measured in Amperes (A). A common voltage source is a chemical battery, which is an example of a direct-current power source (DC). It provides electrons with a fixed amount of energy (i.e. a fixed voltage) through a chemical reaction within a battery. Resistance is a value that describes how easy for electrons to move in a material when voltage is applied. Resistors are devices that can be added to the circuit to impede current flow.
Ohm's Law
There are a few ways to calculate the required values for each component, however one of the basic ways of calculating them is Ohm's law.
The relationship that represents the relationship between voltage, current, and resistance is ohm’s law, represented by: V=IR. The power source in a circuit determines the voltage supplied and the current available. The connected components will draw current from the power source.
There are three arrangements of circuits, series, parallel, and combination.
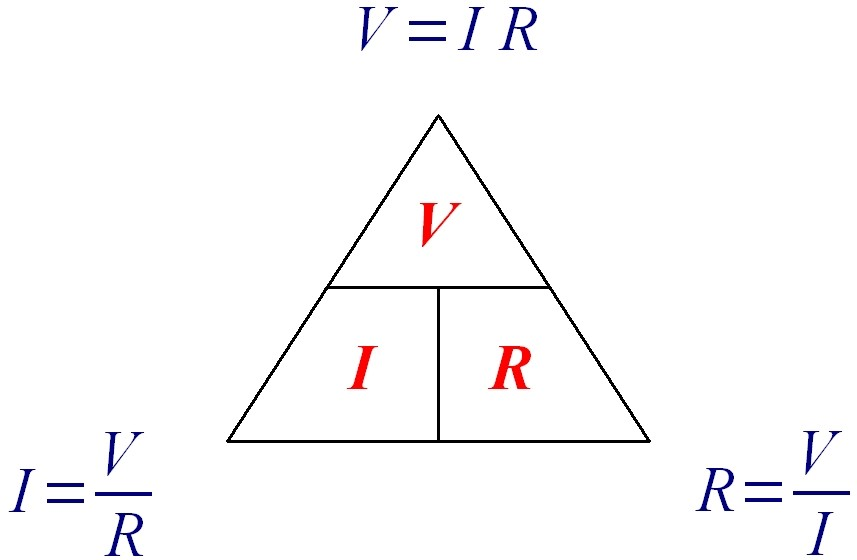
Series Circuits
In a series circuit, the amount of current flowing is the same at all points in the circuit, whereas the voltage supplied by the battery is equal to the voltage drop across each component. A series circuit has only one path for electricity to flow, so if any component fails in the circuit, all other components will also stop operating, as the circuit is now open.
Parallel Circuits
In a parallel circuit, the voltage is the same in all branches, whereas the current is different in each branch.
Introduction to Electronic Components
Breadboard
A breadboard is used to prototype a temporary circuit. The user can build, test and analyze a circuit without any permanent connections. It is made up of terminal strips and power rails. The terminal strips are used to hold any number of components in place and make electrical connections in a horizontal row. The power rails are the long vertical strips and are used to facilitate power (+) and ground (-) connections by placing them all in one column.
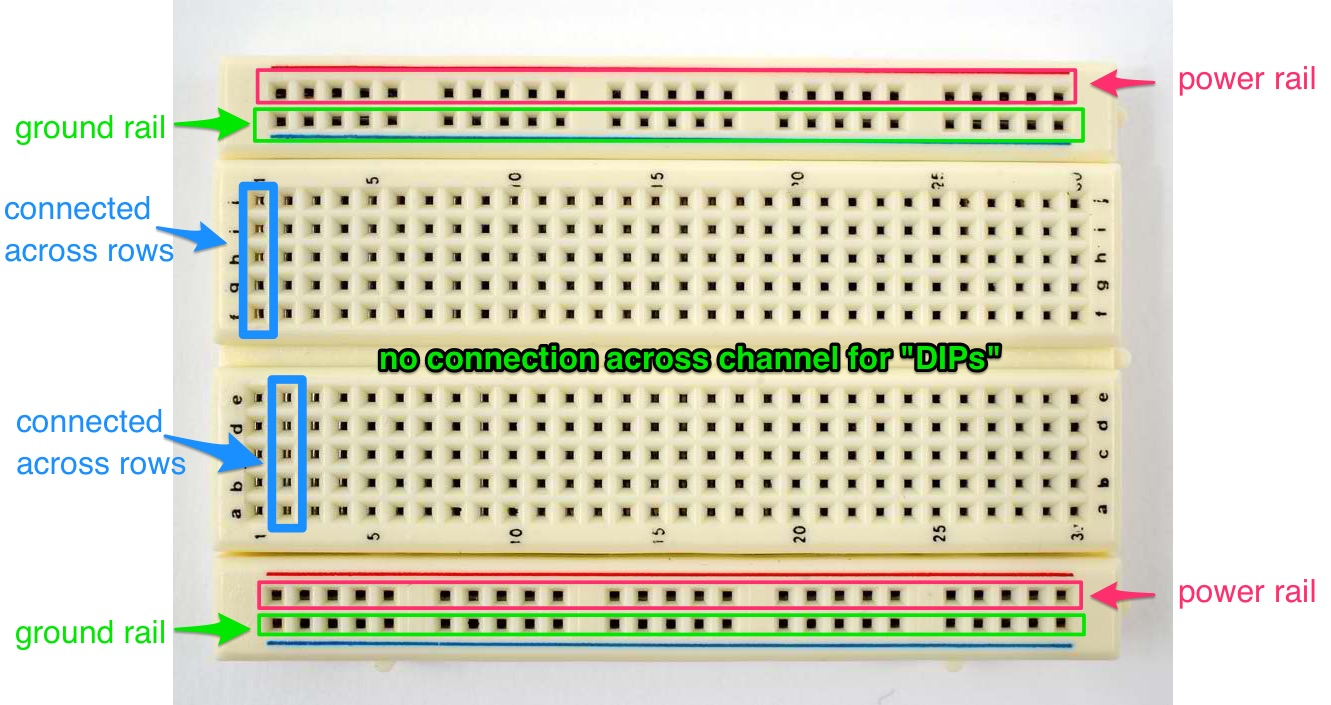
LED
A Light Emitting Diode, or LED, is a semiconductor device that lights up when an electric current passes through it. They come in many different colours and shapes, and are very versatile. LEDs are diodes, which means that current flows through the element in only one way, from the positive to the negative end. On an LED, the cathode end can be identified by either a flat edge on the body, or as the shorter leg. As such, the anode is the other end (the longer leg on the LED).
Pushbutton
A pushbutton is an electronic switch component that completes the circuit only when pressed. In an electric circuit, electricity needs to flow continuously through the circuit in order for all parts to function. The pushbutton interrupts this circuit and forms a gap, so that electricity doesn't flow to the other side of the pushbutton. When the pushbutton is pressed. a small spring is activated that is made of conducting material so that electricity flows through the spring to the other side of the pushbutton.
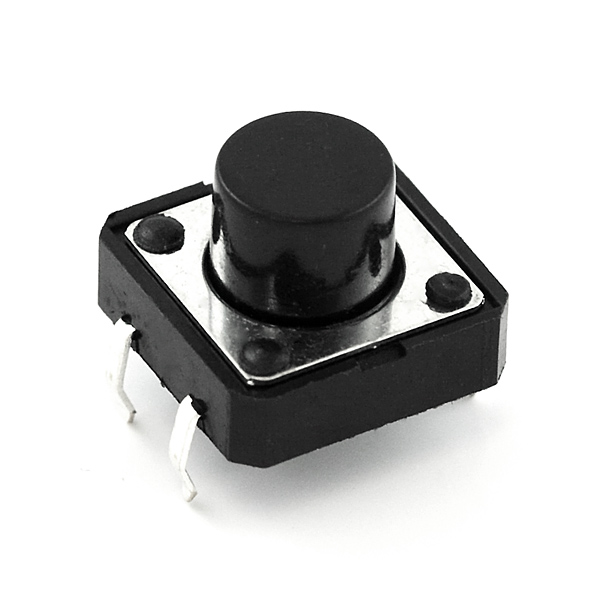
Resistor
A resistor is an electrical component which creates electrical impedance, or resistance to current flow. The amount of resistance a resistor provides can be read from the bands of colour on the resistor, which are read left to right. For four bands resistors, the first and second bands represent digits, while the third band represents a multiplier to multiply the digits of the first and second band by. The fourth band is the tolerance, it represents how much the resistor may deviate from the value indicated by the bands. The value of the resistor can also be determined using the ohmic function of a multimeter.
Resistors are often used in series with components to reduce the amount of current flowing through a circuit, often to protect components rated for lower current amounts. Pull-up and pull-down resistors are used to bias an input on the Arduino to be either HIGH or LOW respectively. This needs to be done as the resting level of the input isn’t necessarily 0. This is especially useful when working with sensors that have an analog output.
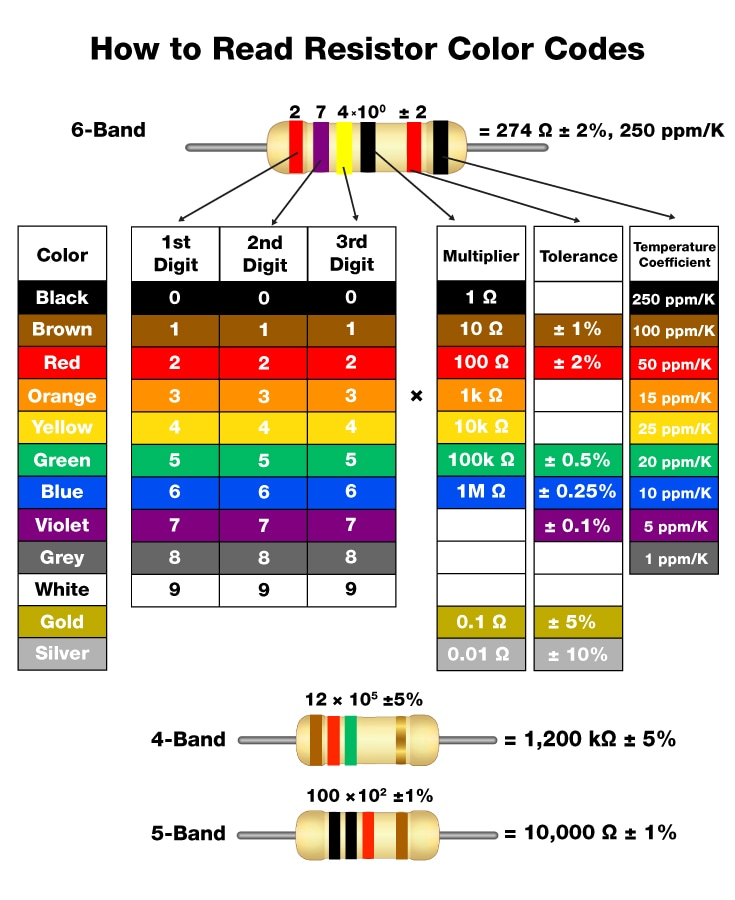
Introduction to Sensors
Sensors enable the microcontroller to sense the surrounding environment. Many sensors exist on the market, including but not limited to buttons, temperature sensors, pressure sensors, photoresistors, humidity and moisture, and many more. The output of the sensor (a voltage) changes based on the measured environment properties, and sends that signal over to the Arduino board.
Different sensors will use different voltage levels and different methods of communication with microcontrollers. It is always important to read their datasheet and make sure they are compatible with your system before continuing or design your system around the sensor specifications.
Online Simulators
Tinkercad is an online platform that enables users to virtually model 3D designs and circuits. Its user friendly interface and functionality encourages students and hobbyists to investigate the functionality of the Arduino boards virtually, as well as start building their designs. To sign up, create an account on: https://www.tinkercad.com, or alternatively, login with google, facebook etc…
Once your account is created, the user can navigate to the “circuits” tab in the left-hand menu, and click on “ Create new circuit”. This opens a new tab, where the available circuit components can be viewed in the right-hand menu.
To start putting a circuit together, the user can drag the components from the library and place it in the “building zone”, noted above. In addition, note the “Code” button in the top bar; this button enables the users to program the Arduino to perform specific functions. To start the simulation, the “start simulation” is available in the top tab.
For more information refer to the following resources: